When getting started with Shopware 6 development, creating a new CLI command is one of the first scenarios you could / should bump to it. This free lesson - part of our Shopware 6 Backend Development training - helps you to get started.
To add a new CLI command to the output of bin/console
, we will need to do two things: We need to create a new Command class in PHP and we need to define that Command class as a service.
Command class
The PHP class is fairly simple: It extends from the Symfony Command
class and needs to implement two methods configure()
and execute()
: The configure()
method is executed whenever we run the bin/console
command without any additional parameters. The name and description that we add here will be added to the output of bin/console
. And once we execute the command itself (in this case bin/console example
), the execute()
method is run.
File src/Command/ExampleCommand.php
namespace Swag\Example\Command;
use Symfony\Component\Console\Input\InputInterface;
use Symfony\Component\Console\Output\OutputInterface;
use Symfony\Component\Console\Command\Command;
class ExampleCommand extends Command
{
protected function configure(): void
{
$this->setName('example')->setDescription('Simple example');
}
protected function execute(
InputInterface $input,
OutputInterface $output): int {
$output->writeln('Hello example');
return 0;
}
}
There is much more possible with the Symfony console: For instance, we can ask the user for input, run through a wizard-like questionnaire and display data in tables. It is best to refer to the Symfony documentation for full details.
Returning a number from the execute()
method is not mandatory ... yet. Symfony 5 makes it mandatory, so therefore it is best practice to add it anyway: A simple 0
for success and something else (nonzero) for failure.
Alternative configuration
Instead of overriding the configure()
method, you could also set $defaultName
and $defaultDescription
:
protected static $defaultName = 'user:list';
protected static $defaultDescription = 'Show a listing of all current users';
Adding options and arguments can only be done by the configure()
method.
If your plugin is going to offer multiple commands, it might be nice to group them together by separating the group name and the command name using a colon, like user:list
.
Defining the command
The PHP class that we created earlier still needs to be registered. To do this, we add a new entry to the services.xml
file to create a new service with an id
that refers back to our namespaced class. And this service is then tagged with console.command
. Through this tag, the Symfony framework is automatically able to detect this class and add it to the console.
File src/Resources/config/services.xml
:
<?xml version="1.0" encoding="UTF-8" ?>
<container xmlns="http://symfony.com/schema/dic/services"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://symfony.com/schema/dic/services http://symfony.com/schema/dic/services/services-1.0.xsd">
<services>
<service id="Swag\Example\Command\ExampleCommand">
<tag name="console.command"/>
</service>
</services>
</container>
Do make sure that the class is not starting with a forward slash, for instance while copying the class name by reference.
Once done, we need to refresh the cache (bin/console cache:clean
) and then the command should be visible and executable.
By intent, we have not added anything useful to the command yet, so you can see that creating the command only involves two simple steps: The class and the service definition. However, most likely, you will want to add dependencies to this class to get actual work done. This is covered in the next lesson.
Like this lesson? We got plenty more. Sign-up now for our online trainings and get yourself started properly
About the author
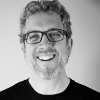
Jisse Reitsma is the founder of Yireo, extension developer, developer trainer and 3x Magento Master. His passion is for technology and open source. And he loves talking as well.